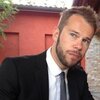
Joris De Groot
For a project estimate I did some research in loading in a pdf template, adding some images to it and saving it to a new pdf and preview images per page. The technologies I used are FPDF, FPDI and Imagemagick with Ghostscript, all together in php on a local Windows XAMPP server environment. Here's how it went:
XAMPP already installed so no work with that.
Installation of Imagemagick
As I have a x64 Windows 7 machine I first got the Win64 dynamic at 16 bits-per-pixel - ImageMagick-6.7.4-6-Q16-windows-x64-dll.exe, here which didn't seem to work. Apparently the official version is compiled in a way it's incompatible with my version of XAMPP, but the dll downloaded from here got it working. Installed in XAMPP's php/ext directory, added extension=php_imagick_ts.dll to XAMPP's php/php.ini!!! Should add something about installing imagemagick itself (not the dll)
Installation of GhostScript Just download and install the exe.
Installation of FPDF & FPDI Downloaded and installed FPDF & FPDI to the fpdf/
directory of the webapp's root.
I generated a sample pdf to put the images in with Photoshop, ugly as hell, but it should be doing the trick. Be sure to save the pdf with a compression version Ghostscript can handle, just to be sure I used the lowest Adobe PS5.1 offered me: 1.4.
First of the requires:
require_once('fpdf/fpdf.php'); require_once('fpdf/fpdi.php');
Then we make a new FPDI object and load the template pdf.
$pagecount = $pdf->setSourceFile('template.pdf');
Then we run through the pages and add the images. This template pdf contains only one page, and i add only two images, so you should probably set an array or something which add specific images to specific pages and coordinates (these are hardcoded in this example).
for ($i=1; $i <= $pagecount; $i++) { $tplidx = $pdf->ImportPage($i); $pdf->Image('picture_1.jpg',10,8,33); $pdf->Image('picture_2.jpg',10,8,33); $pdf->addPage(); $pdf->useTemplate($tplidx,0,0,0); }
Next, the output is sent to a new pdf file.
$pdf->Output('new.pdf', 'F');
And after that, the preview images are generated using imagemagick.
$magick_dir = 'D:\xampp\ImageMagick-6.7.4-Q8' ; $send_cmd=$magick_dir .'\convert newpdf.pdf -resize x100 test.jpg' ; $result = shell_exec($send_cmd) ;
This is my full PHP code:
setSourceFile('input/template.pdf'); for ($i=1; $i <= $pagecount; $i++) { $tplidx = $pdf->ImportPage($i); $pdf->addPage(); $pdf->useTemplate($tplidx,0,0,0); $pdf->Image('input/picture_1.jpg',62,40,-300); $pdf->Image('input/picture_2.jpg',62,180,-300); } $pdf->Output('new.pdf', 'F'); $magick_dir = 'D:\xampp\ImageMagick-6.7.4-Q8' ; $send_cmd=$magick_dir .'\convert output/new.pdf -resize x100 output/preview.jpg' ; $result = shell_exec($send_cmd) ;